Deploying Self-Hosted GlitchTip for Error Tracking (Sentry alternative)
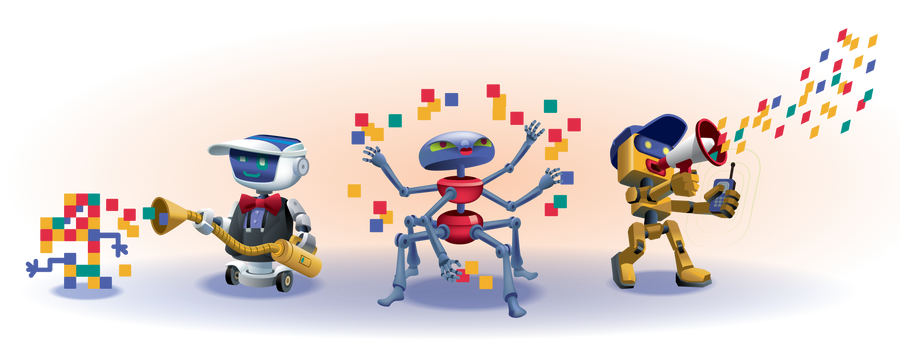
Error tracking is a critical part of software development, allowing you to identify and resolve issues in your applications quickly. GlitchTip is an open-source error tracking system that simplifies monitoring and managing errors in your software.
In this tutorial, we will guide you through deploying a self-hosted GlitchTip instance without Docker, securing it with Nginx as a reverse proxy, setting up Let's Encrypt for SSL/TLS encryption, and managing GlitchTip using systems.
Additionally, we'll provide examples of how to implement error tracking for both a Node.js application and a Python script.
Prerequisites
Before we get started, ensure you have the following prerequisites:
- A server or cloud instance where you can host GlitchTip. You can use a virtual machine, a physical server, or a cloud-based service like AWS, DigitalOcean, or Google Cloud.
- A domain or subdomain pointing to your server's IP address. You'll use this domain for accessing your GlitchTip instance and obtaining Let's Encrypt SSL/TLS certificates.
- Basic knowledge of Linux command-line operations, as we'll be using a Linux-based server for this tutorial.
- Node.js and Python installed on your local development machine for the application and script examples.
- Step 1: Setting Up GlitchTip
Install glitchtip on linux
SSH into your server or access it via a web console.
Update the package list to ensure you have the latest information about available packages and Install the required dependencies :
sudo apt update
sudo apt install -y python3-pip python3-venv libpq-dev build-essential
Create a directory to store the GlitchTip application
sudo mkdir /opt/glitchtip
Change ownership of the directory to your user:
sudo chown -R your_username:your_username /opt/glitchtip
Replace your_username
with your actual username.
Clone the GlitchTip repository from GitHub:
git clone https://github.com/glitchtip/glitchtip.git /opt/glitchtip
Create a virtual environment for GlitchTip:
python3 -m venv venv
Activate the virtual environment:
source venv/bin/activate
Install the required Python packages:
pip install -r requirements.txt
Create a configuration file for GlitchTip:
cp config.example.py config.py
Open the config.py
file in a text editor and configure the following settings:SECRET_KEY
: Replace with a strong, unique secret key.DEBUG
: Set to False
for production use.ALLOWED_HOSTS
: Add your server's domain or IP address.EMAIL_*
settings: Configure email notifications if desired.
Database Setup
GlitchTip uses PostgreSQL as its database. You can install it and set it up as follows:
Install PostgreSQL:
sudo apt install -y postgresql postgresql-contrib
Log in to the PostgreSQL interactive terminal as the postgres
user:
sudo -i -u postgres
Create a PostgreSQL user and database for GlitchTip (replace glitchtipuser
and glitchtipdb
with your preferred names):
createuser glitchtipuser;
createdb glitchtipdb;
Set a password for the GlitchTip PostgreSQL user:
psql
\password glitchtipuser
Follow the prompts to set the password.
Exit the PostgreSQL interactive terminal:
\q
exit
Initialize GlitchTip
Run the database migrations to set up the GlitchTip database:
cd /opt/glitchtip
flask db upgrade
Create a superuser account for the GlitchTip web interface:
flask users create
Follow the prompts to create the superuser account.
Install & configure Nginx for glitchtip
If Nginx is not already installed on your server, you can install it using the following command:
sudo apt install -y nginx
Create an Nginx server block configuration file for GlitchTip. Replace your_domain_or_ip
with your server's domain or IP address.
sudo nano /etc/nginx/sites-available/glitchtip
Add the following configuration to the file:
server {
listen 80;
server_name your_domain_or_ip;
location / {
proxy_pass http://localhost:8000;
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
}
location /static/ {
alias /opt/glitchtip/app/static/;
}
location /uploads/ {
alias /opt/glitchtip/uploads/;
}
location /errors/ {
alias /opt/glitchtip/app/errors/;
}
location /media/ {
alias /opt/glitchtip/app/media/;
}
location ~ /\.well-known/acme-challenge {
allow all;
}
}
Save and exit the text editor.
Create a symbolic link to enable the Nginx server block configuration:
sudo ln -s /etc/nginx/sites-available/glitchtip /etc/nginx/sites-enabled/
Test the Nginx configuration for syntax errors:
sudo nginx -t
If there are no errors, reload Nginx to apply the new configuration:
sudo systemctl reload nginx
Install Certbot to configure SSL/TLS
If Certbot is not already installed on your server, you can install it using the following command:
sudo apt install -y certbot python3-certbot-nginx
Run the Certbot command to obtain SSL/TLS certificates for your domain:
sudo certbot --nginx -d your_domain
Follow the prompts to configure Certbot and generate the certificates. Certbot will automatically update the Nginx configuration to use HTTPS.
Running GlitchTip with systemd
To run GlitchTip as a systemd service, you'll create a systemd service unit file.
sudo nano /etc/systemd/system/glitchtip.service
Add the following content to the file:
[Unit]
Description=GlitchTip Error Tracking Service
After=network.target
[Service]
User=your_username
Group=your_username
WorkingDirectory=/opt/glitchtip
ExecStart=/opt/glitchtip/venv/bin/gunicorn -w 4 -b 0.0.0.0:8000 "glitchtip:create_app()"
[Install]
WantedBy=multi-user.target
Replace your_username
with your actual username.
Save and exit the text editor.
Start the GlitchTip service and enable it to start on boot:
sudo systemctl start glitchtip
sudo systemctl enable glitchtip
Check the status of the GlitchTip service to ensure it's running:
sudo systemctl status glitchtip
Visit https://your_domain_or_ip
in your web browser to access the GlitchTip web interface. You should see the initial setup page.
Setup GlitchTip with Node.js Application
For Node.js applications, you can use the glitchtip-node
npm package to integrate error tracking with GlitchTip. Here's an example of how to do it:
Install the glitchtip-node
package in your Node.js project:
npm install glitchtip-node
In your Node.js application, require and initialize GlitchTip with your GlitchTip server URL and project API key:
const glitchtip = require('glitchtip-node');
glitchtip.initialize({
serverUrl: 'https://your_domain_or_ip',
projectApiKey: 'your_project_api_key',
});
- Replace
your_domain_or_ip
with your GlitchTip server's domain or IP address andyour_project_api_key
with your actual project's API key from the GlitchTip web interface. - Now, whenever an error occurs in your Node.js application, GlitchTip will capture and report it to your self-hosted instance over HTTPS.
Setup GlitchTip with python Application
For Python scripts, you can use the glitchtip
Python package to integrate error tracking with GlitchTip. Here's an example of how to do it:
Install the glitchtip
package using pip:
pip install glitchtip
In your Python script, import and initialize GlitchTip with your GlitchTip server URL and project API key:
import glitchtip
glitchtip.init(
server_url='https://your_domain_or_ip',
project_api_key='your_project_api_key'
)
- Replace
your_domain_or_ip
with your GlitchTip server's domain or IP address andyour_project_api_key
with your actual project's API key from the GlitchTip web interface. - Now, whenever an exception occurs in your Python script, GlitchTip will capture and report it to your self-hosted instance over HTTPS.
Conclusion
You have successfully deployed a self-hosted GlitchTip instance for error tracking, secured it with Nginx and Let's Encrypt, and managed it using systems.
Additionally, you've integrated it with both a Node.js application and a Python script. GlitchTip provides valuable insights into your application's errors, helping you diagnose and resolve issues more efficiently.
Regularly review and address the errors reported in GlitchTip to improve the reliability and performance of your software.